Substituting Event Data into Messages
Mobile app developers often need to send dynamic content messages to their users. For example, transactional messages initiated from the backend: order confirmation, delivery, etc.
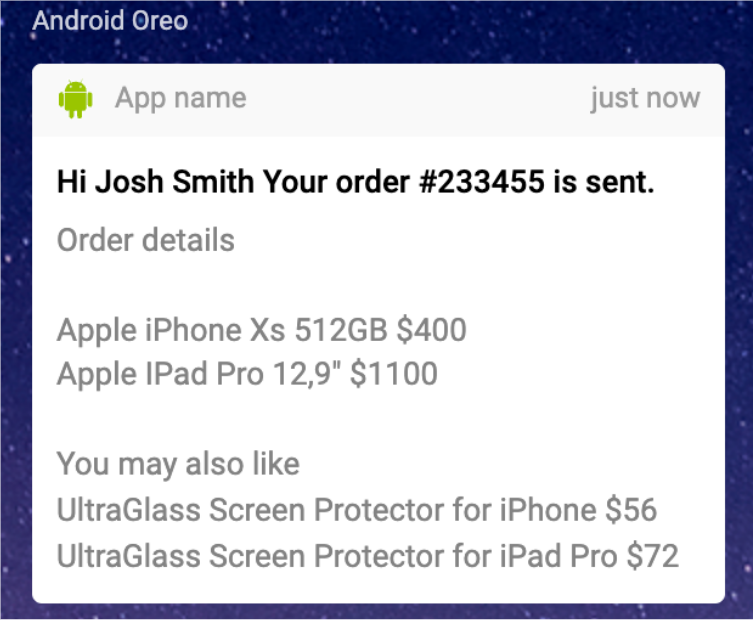
We recommend using Generate event API method to send custom backend events to Reteno. For more information on sending orders data, please read the instructions >
Let's consider in practice how to substitute data from events to a message.
Note
The message with dynamic event content must participate in the workflow triggered by the corresponding event.
See the following instructions to learn how to launch a triggered campaign on event >
Event Data Types
An event can contain different data types, which are substituted differently in the message:
- Simple data (strings and numbers not included in the object or array)
- Object data
- Array data
Let's consider how to transfer these types of data to the message, using the following request as an example:
{
"eventTypeKey": "data_for_message",
"keyValue": "[email protected]",
"params":
[
{
"name": "EmailAddress",
"value": "[email protected]"
},
{
"name": "externalOrderId",
"value": 20233
},
{
"name": "sellerName",
"value": "Shop Tea"
},
{
"name": "object",
"value":
{
"title": "Black Tea",
"text": "Tea from the Tonganagaon plantation in India",
"img": "https://pics.site.com/repository/home/1/common/images/1576074904732.jpg",
"price": "$11.05",
"link": "https://site.com"
}
},
{
"name": "array",
"value":
{
"array_items":
[
{
"title": "Black Tea",
"text": "Tea from the Tonganagaon plantation in India",
"img": "https://pics.site.com/repository/home/1/common/images/1576074904732.jpg",
"price": "$11.05"
},
{
"title": "Green Tea",
"text": "Tea from the Indian region of Darjeeling",
"img": "https://pics.site.com/repository/home/1/common/images/1576074904060.jpg",
"price": "$10.20"
},
{
"title": "Herbal tea",
"text": "Peach, rosehip, and apple, spring flavor.",
"img": "https://pics.site.com/repository/home/1/common/images/1576074902892.jpg",
"price": "$15.00"
}
]
}
}
]
}
Substituting simple data from event
We have "externalOrderId" and "sellerName" fields with 20233 and "Shop Tea" values. These string and number are not included in the object or array.
To substitute values to the message:
- In the message template, use $data.get('externalOrderId') and $data.get('sellerName') velocity constructions, where externalOrderId and sellerName are field names from the request.
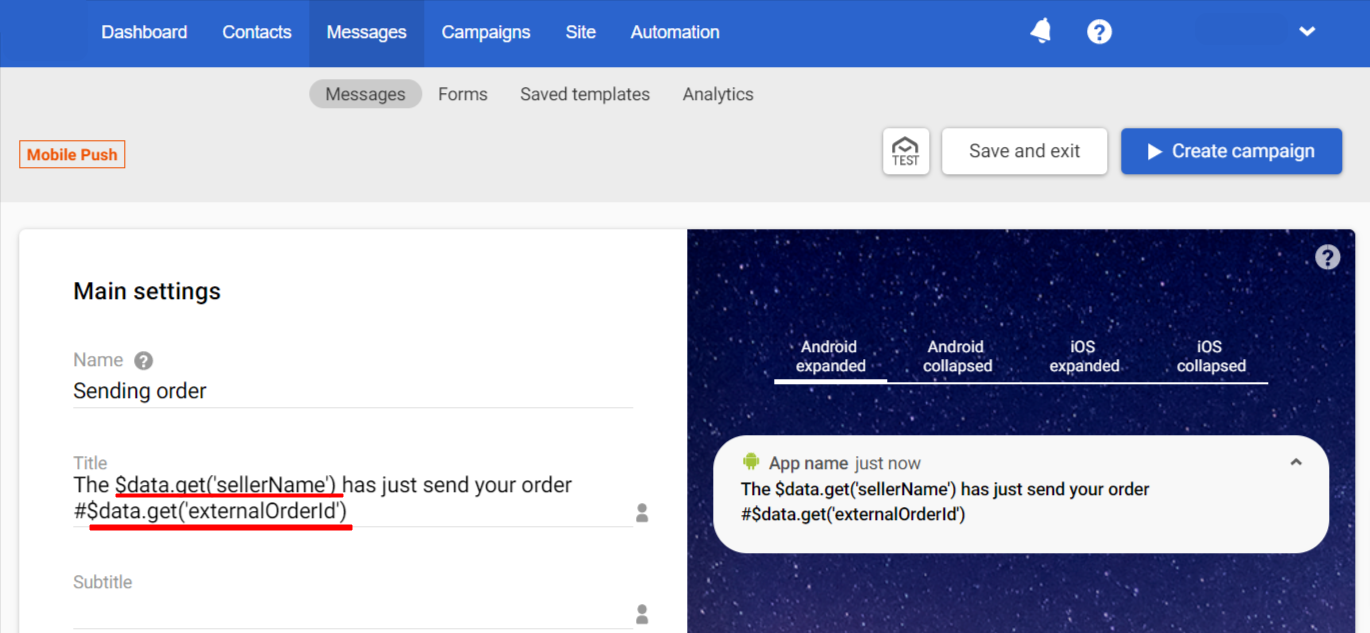
The user who initialized the event will receive the message:
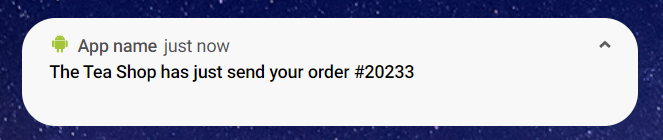
Substituting object data from event
In the example, the object is the data of one product:
{
"name": "object",
"value":
{
"title": "Black Tea",
"text": "Tea from the Tonganagaon plantation in India",
"img": "https://pics.site.com/repository/home/1/common/images/1576074904732.jpg",
"price": "$11.05",
"link": "https://site.com"
}
}
To access object data:
- Select the message block in the workflow and specify the object name in the JSON field (in this request, ${object}).
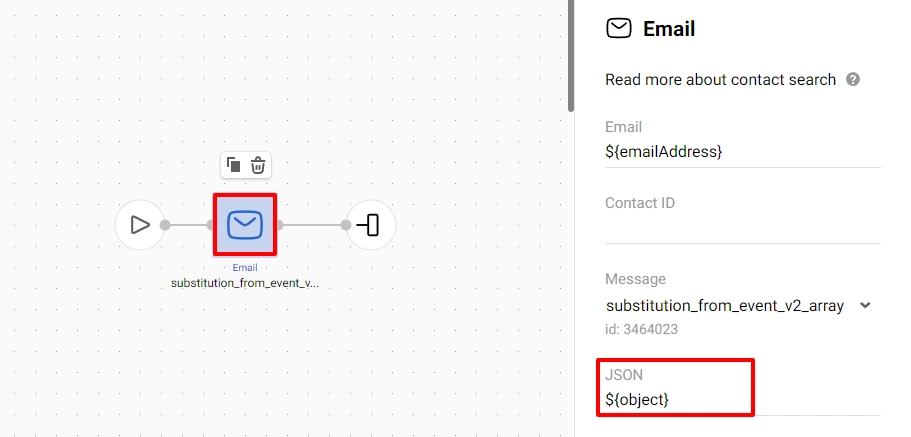
- In the message template, specify the velocity variables from the object in the appropriate fields:
- $data.get('title')
- $data.get('text')
- $data.get('img')
- $data.get('price')
- $data.get('link')
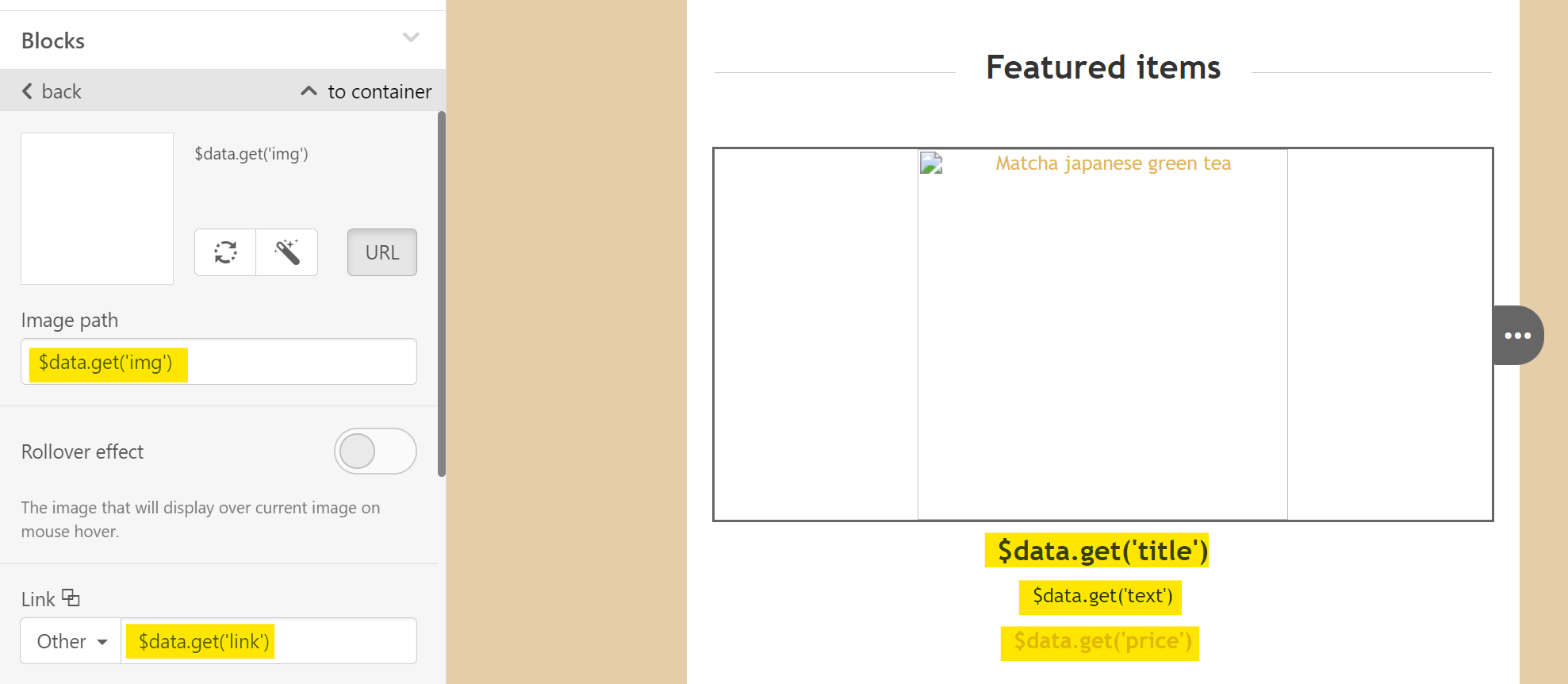
The email will look like this:
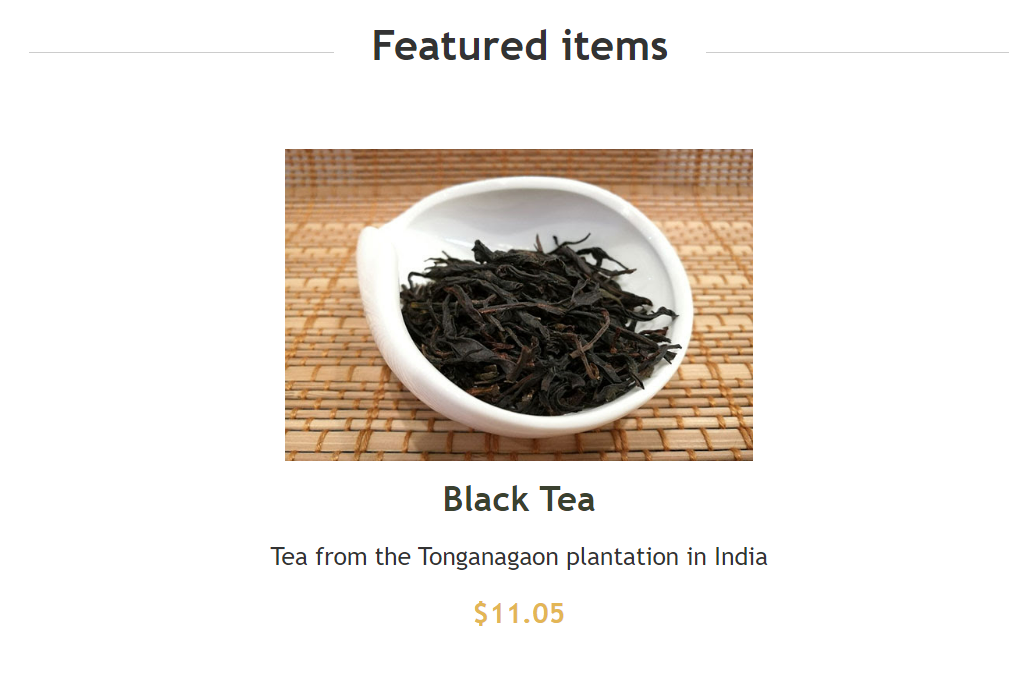
Sending object data covers most needs for channels like SMS, Web Push, Mobile Push, Viber, and App Inbox.
To send more data, use an array.
Substituting array data from event
In the example, data about several products are transferred in the array:
{
"name": "array",
"value":
{
"array_items":
[
{
"title": "Black Tea",
"text": "Tea from the Tonganagaon plantation in India",
"img": "https://pics.site.com/repository/home/1/common/images/1576074904732.jpg",
"price": "$11.05"
},
{
"title": "Green Tea",
"text": "Tea from the Indian region of Darjeeling",
"img": "https://pics.site.com/repository/home/1/common/images/1576074904060.jpg",
"price": "$10.20"
},
{
"title": "Herbal tea",
"text": "Peach, rosehip, and apple, spring flavor.",
"img": "https://pics.site.com/repository/home/1/common/images/1576074902892.jpg",
"price": "$15.00"
}
]
}
}
To access array data:
- Select the message block in the workflow and enter the array name in the JSON field (in our example it is ${array}).
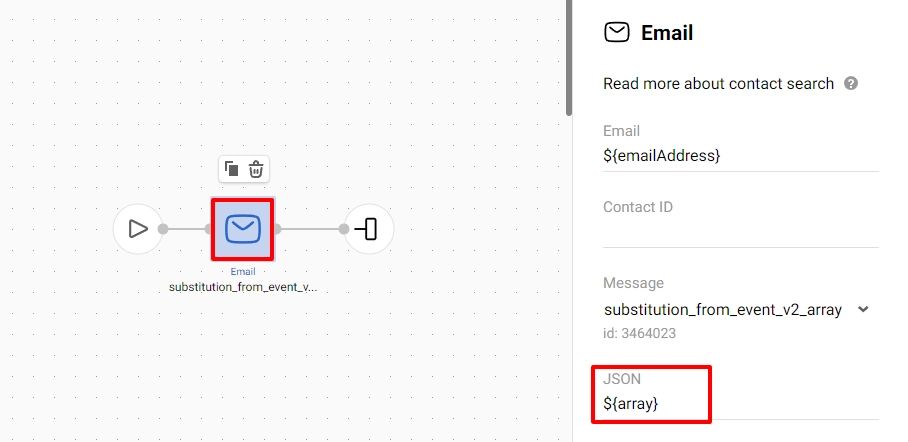
- In the email editor, highlight the appropriate strip, open the code, and add the velocity structure with the _#foreach _loop:
<!-- #foreach( $item in $data.get('array_items') ) -->
// html content
<!-- #end -->
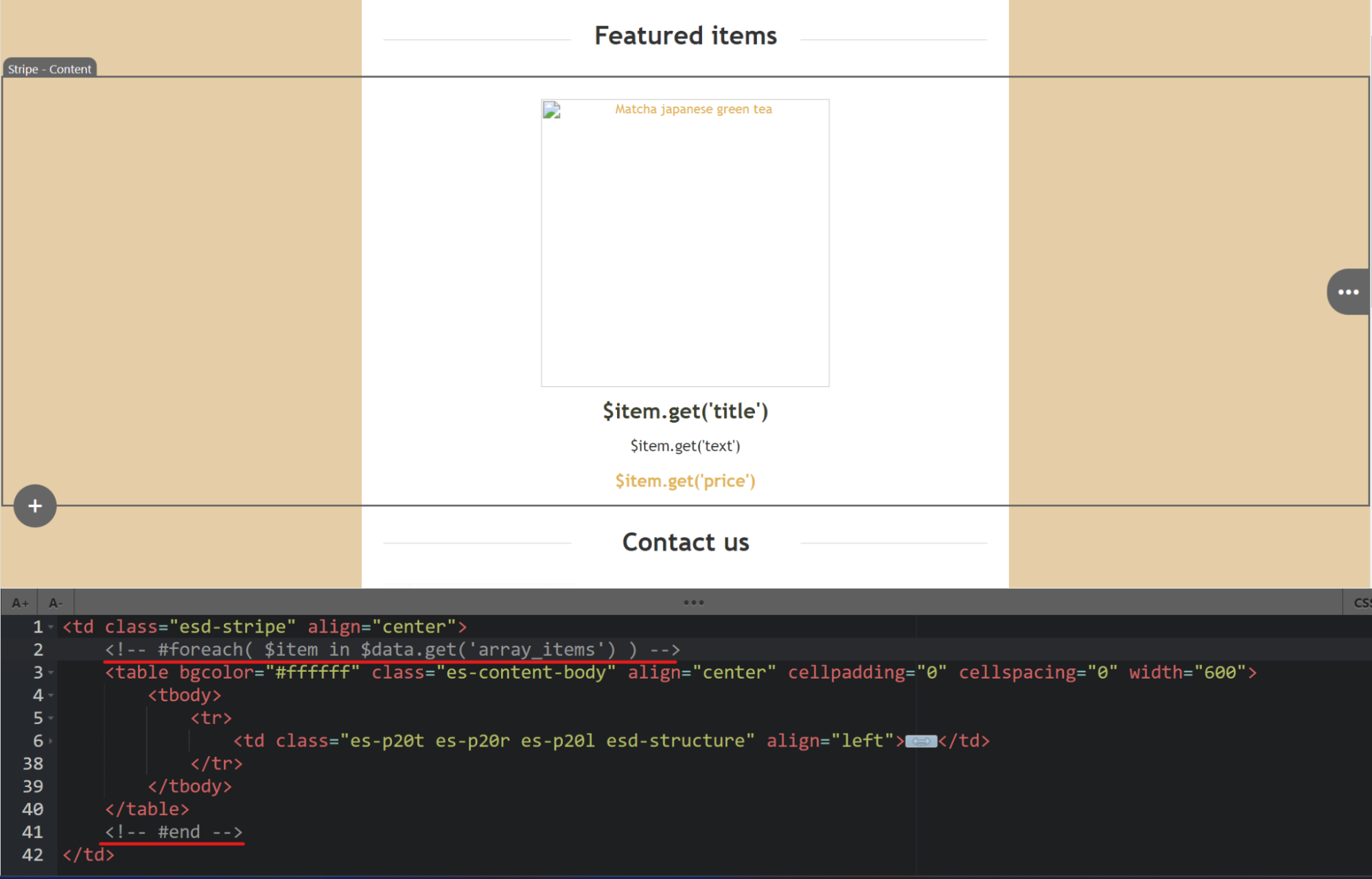
The loop sequentially goes through the array elements, placing the data in the intermediate object $items (arbitrary name), which is used to substitute data into the message.
- Specify the velocity variables from the array in the appropriate places of the temlate:
- $item.get('title')
- $item.get('text')
- $item.get('img')
- $item.get('price')
- $item.get('link')
The email will look like this:
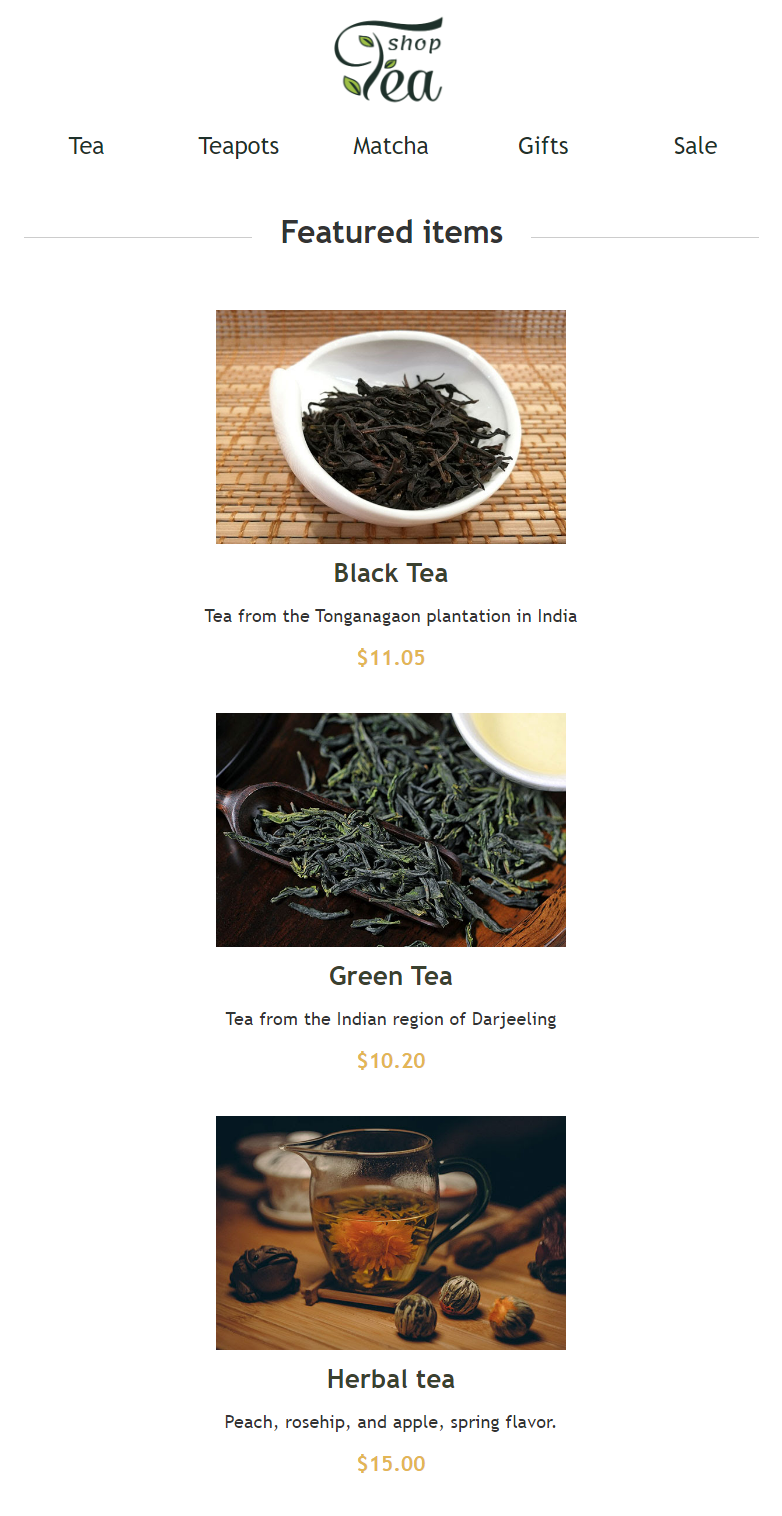
Note
The mobile push notification will be displayed differently on different devices, but we recommend writing at most 40 characters. That’s why it is not always rational to display the entire contents of the array using the foreach loop.
Instead, you can refer to a specific array element. Then velocity construction for the array will look like this: $!data.get('array_items').get(0).get(' name'), where the first element of the array is accessed (numbered from 0).
Updated 12 months ago